Recently, I started a side project where I was working with Facebook Lead Generations Ads. My idea was to get a list of names and email addresses that had a specific interest and then send them an automated email. So first I connected my Facebook ads to a free HubSpot account and then I wanted to use the built-in mailing feature from Hubspot. But guess what, you have to wait for a few days before Hubspot approves your request to send emails. The impatient person that I am, I started thinking that I could solve this problem with Python. So here, I will explain to you how to send automated emails using Python.
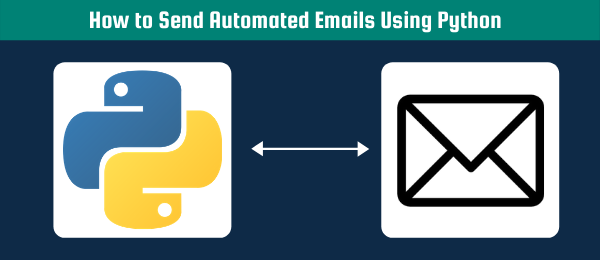
Time needed: 10 minutes
How to Send Automated Emails Using Python
- Basic Automated Email with smtplib
You can send emails in Python using smtplib
- Personalise your Automated Email
Using placeholders and the replace function in Python, you can personalise each email
- Add a PDF document to your automated email
We can even add attachments such as PDF documents to the automated email
- Loop over Input File (CSV/Excel)
We can loop over each row of a CSV file or Excel file to populate dynamic values to send emails to different addresses
Input for Automated Emails
Well als you can imagine, you will need some kind of list of email addresses. If you want to personalise them, you will also need extra information such as first and last names. Luckily for me, my Facebook Ads were collecting emails and names so I had a simple table with three columns:
- First Name
- Last Name
- Email Address
As I am trying to send these automated emails using Python, I exported an excel file from Hubspot and that would be my input. If you have any type of excel or CSV file with each row being a person and at least one column with email address, then you are ready to go.
Approach for Automated Emails
We are going to use smtplib, a Python module specifically made to send email addresses using SMTP. Just for the more technical people around there, SMPT is Simple Mail Transfer Protocol and it involves sending emails. However, IMAP is Internet Access Message Protocol and involves sending emails. Then on top of that, there is another technology called POP3 which stands for Post Office Protocol. This one involves receiving emails as well, but instead of keeping the email on a server, POP3 will delete it from a server. Therefore, an email is only stored on the device that downloaded it.
Step 1. Basic Automated Email with smtplib
If you look at the code below it is all really self-explanatory. we are using MIMEMultipart to add information about our email to the msg variable. For those that didn’t know, emails nowadays can be written in HTML instead of plain text. In a way, an email is just a small webpage that is shown to a user (with of course some limitations). Therefore, I am showing a method for sending an HTML email. We are using triple quotations so we can add enters and two kinds of quotations inside our email without any problems.
If you run this code below, Python will start connecting to your email servers using your credentials and then send an email address on behalf of you.
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
sender = "sender@example.com" # replace with sender's email address
receiver = "receiver@example.com" # replace with receiver's email address
password = "SECRETPASSWORD" # replace with your password
host = 'mail.axc.nl' # replace with your host, e.g. gmail = smtp.gmail.com
port = 465 # replace with your port, e.g. gmail = 465
s = smtplib.SMTP_SSL(host, port)
#s.set_debuglevel(1)
s.login(me, password)
html_mail = """<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Thank you for your Interest</title>
<h1> Title of Email </h1>
<p> Content of Email </p>
"""
msg = MIMEMultipart('alternative')
msg['Subject'] = f"Test Automated Email" # replace with your subject
msg['From'] = sender
msg['To'] = receiver
html = html_mail
part2 = MIMEText(html, 'html')
msg.attach(part2)
s.sendmail(me, you, msg.as_string())
s.quit()
Step 2. Personalise your automated email
If you are going to send automated emails, you might want to add some personalization in there. We are going to add dynamic values using a really simple method. We add placeholders inside our HTML variable, which we then replace with the dynamic value.
In the example below I have added a placeholder {{first_name}}. In the line below we are replacing that value using .replace. I have added a simple for loop that loops over some first_names. In real life, you will have a column in your CSV file which you can use to populate the first_name value.
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
sender = "sender@example.com" # replace with sender's email address
receiver = "receiver@example.com" # replace with receiver's email address
password = "SECRETPASSWORD" # replace with your password
host = 'mail.axc.nl' # replace with your host, e.g. gmail = smtp.gmail.com
port = 465 # replace with your port, e.g. gmail = 465
s = smtplib.SMTP_SSL(host, port)
#s.set_debuglevel(1)
s.login(me, password)
first_names = ["Armando", "John"]
for first_name in first_names:
html_mail = """<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1
<title>{{first_name}}, Thank you for your Interest</title>
<h1> Title of Email </h1>
<p> Hi, {{first_name}} </p>
"""
msg = MIMEMultipart('alternative')
msg['Subject'] = f"Test Automated Email" # replace with your subject
msg['From'] = sender
msg['To'] = receiver
html = html_mail
part2 = MIMEText(html, 'html')
msg.attach(part2)
s.sendmail(me, you, msg.as_string())
s.quit()
Step 3 Add a PDF document to your automated email
You can also add attachments with smtplib. In my situation I had to send some vouchers using my Python script.
In the code below I have added four lines of codes to show you how you can add a PDF to your email in Python. You can use different methods, but this one is the only one that does not result in an encoding error.
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
df = pd.read_excel("input.xlsx") # replace with your input file
sender = "sender@example.com" # replace with sender's email address
receiver = "receiver@example.com" # replace with receiver's email address
password = "SECRETPASSWORD" # replace with your password
host = 'mail.axc.nl' # replace with your host, e.g. gmail = smtp.gmail.com
port = 465 # replace with your port, e.g. gmail = 465
s = smtplib.SMTP_SSL(host, port)
#s.set_debuglevel(1)
s.login(me, password)
html_mail = """<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1
<title>Thank you for your Interest</title>
<h1> Title of Email </h1>
<p> Content of Email </p>
"""
msg = MIMEMultipart('alternative')
msg['Subject'] = f"Test Automated Email" # replace with your subject
msg['From'] = sender
msg['To'] = receiver
attachment_path = 'voucher.pdf' # replace with path of your PDF file
with open(attachment_path, "rb") as f:
attach = MIMEApplication(f.read(),_subtype="pdf")
attach.add_header('Content-Disposition','attachment',filename=str(attachment_path))
msg.attach(attach)
html = html_mail
part2 = MIMEText(html, 'html')
msg.attach(part2)
s.sendmail(me, you, msg.as_string())
s.quit()
Step 4. Loop over Input File and Send Automated Emails using Python
As I mentioned at the beginning of this article, we want to use an input file such as a CSV or Excel file. That file contains all our necessary information, and we can loop over the file to populate our placeholders.
In the example below shows how you can loop over your excel file and extract a column value. I know it’s not the most efficient way of looping over an Excel file, but I do think it is one of the most intuitive and easy approaches.
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
df = pd.read_excel("input.xlsx") # replace with your input file
sender = "sender@example.com" # replace with sender's email address
password = "SECRETPASSWORD" # replace with your password
host = 'mail.axc.nl' # replace with your host, e.g. gmail = smtp.gmail.com
port = 465 # replace with your port, e.g. gmail = 465
s = smtplib.SMTP_SSL(host, port)
#s.set_debuglevel(1)
s.login(me, password)
for i, row in df.iterrows():
first_name = row["First Name"]
receiver = row["Email"]
html_mail = """<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1
<title>{{first_name}}, Thank you for your Interest</title>
<h1> Title of Email </h1>
<p> Content of Email </p>
"""
msg = MIMEMultipart('alternative')
msg['Subject'] = f"Test Automated Email" # replace with your subject
msg['From'] = sender
msg['To'] = receiver
attachment_path = 'voucher.pdf' # replace with path of your PDF file
with open(attachment_path, "rb") as f:
attach = MIMEApplication(f.read(),_subtype="pdf")
attach.add_header('Content-Disposition','attachment',filename=str(attachment_path))
msg.attach(attach)
html = html_mail
part2 = MIMEText(html, 'html')
msg.attach(part2)
s.sendmail(me, you, msg.as_string())
s.quit()
I hope you have enjoyed learning how to send automated emails using Python. If you are new to Python, don’t forget to check out my article on how to install Python. If you have any questions, do not hesitate to ask them in the comments below.
What are “me”,”you”? I dont’t understand!
Me = Sender
You = Receiver
But yeah that’s a small error in the code, as those variables have already been defined with a separate name