By default, Selenium creates a new Chrome profile each time you load the Selenium web driver. Technically, this means that all persistent data such as cookies, cache, and internal storage are cleaned. Practically, this means a website will consider you a new user each time you visit a page. This can be very annoying if you are working with a website that has a long login process or complicated reCAPTCHA elements. In this article, I will explain how you can save your Chrome profile for your Selenium web driver in Python.
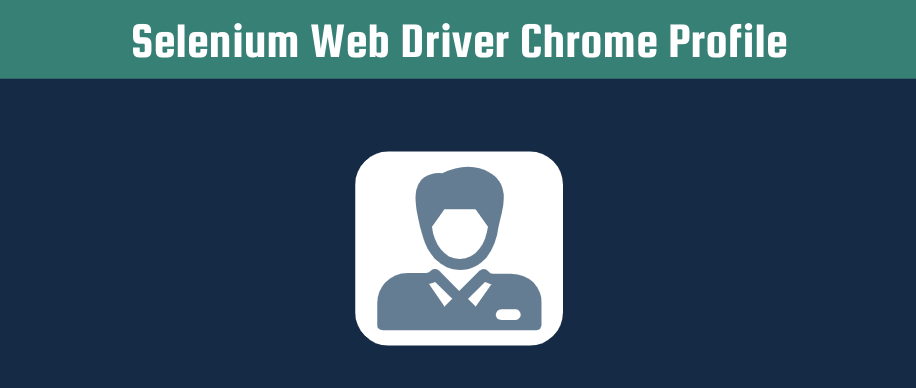
Retrieve Selenium Web Driver Chrome Profile
I assume you already know how to set up your Selenium web driver, but please check my article to find an easy setup for the selenium web driver. Conceptually you can imagine the Selenium driver to be the controller of your browser. The driver will tell your browser what to do and you will be able to load all dynamic elements that are dependent on JavaScript. Your web driver chrome profile contains all the information about your browsing history. Actually, if you are using Chrome at this moment, you already have a Chrome profile that you can use for your Selenium web driver. You can navigate to “chrome://version” to find your Profile Path.
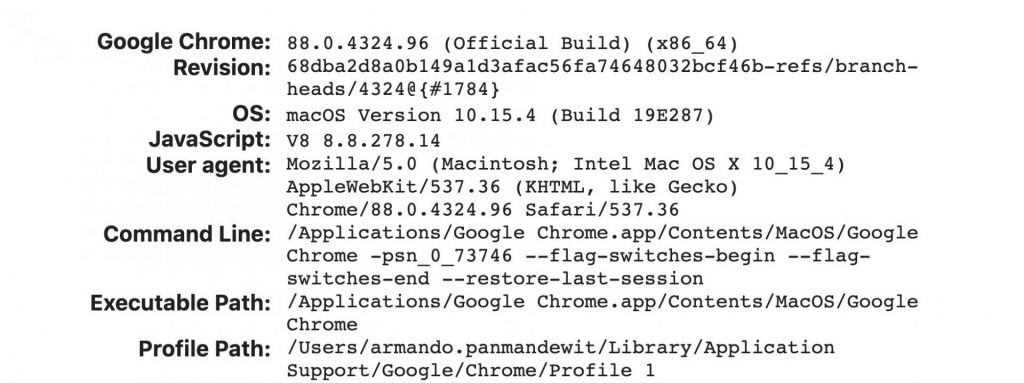
Save Selenium Web Driver Chrome Profile
When using Selenium you can specify a chrome profile path. If that path is empty, your Selenium web driver will create a blank profile. However, you can also move all the contents from your existing profile path mentioned in the previous section. With the following lines of code, you can add an argument to your chrome options. This argument will be loaded when you define your driver.
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
chromeDriverPath = '/users/armando.panmandewit/documents/python/selenium/chromedriver'
userdatadir = '/users/armando.panmandewit/documents/python/selenium/userData'
chromeOptions = webdriver.ChromeOptions()
chromeOptions.add_argument(f"--user-data-dir={userdatadir}") #Path to your chrome profile
driver = webdriver.Chrome(chromeDriverPath, options=chromeOptions)
Each time you run your script, you should close the browser window. Otherwise, you will receive an error that the folder is already in use. If you get another error, please do not hesitate to add this in the comments below.
I hope you better understand the Selenium web driver chrome profile in Python. I always enjoy hearing your feedback, so please leave a message in the comment or contact me on social media.
Pingback: Create a simple web scraper using Selenium - Automation Help
I tried like that and it didn’t work
can you help me;?
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
chromeDriverPath = ‘\Users\edson\Documents\testepython\chromedriver.exe’
userdatadir = ‘\Users\edson\Documents\testepython\userData’
chromeOptions = webdriver.ChromeOptions()
chromeOptions.add_argument(f”–user-data-dir=C:\Users\edson\AppData\Local\Google\Chrome\User Data\Profile 1{userdatadir}”)
driver = webdriver.Chrome(chromeDriverPath, options=chromeOptions)
Hi Edson,
Thank you for reaching out! I would love to help out.
Could you add a bit more information about the error message that you are receiving?
One issue I seem to encounter often is that you need to close your Selenium browser after running your script. Otherwise, it will try to open two browsers that are using the same profile and that is not allowed.
Best Regards,
Armando