Are you trying to understand How to use implicit wait in Selenium web driver? In this article, I will dive deeper into how you can make sure you wait for certain elements when you are running a Selenium web driver. Whether you are a complete newcomer to the world of Python, or even if you have written many lines of code in Python, I will try to make this article understandable for anyone.
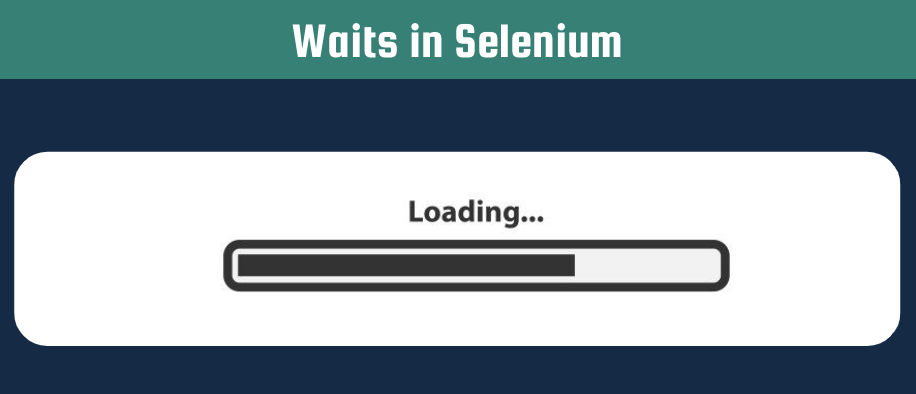
Selenium web driver
The Selenium web driver allows you to automate QA processes, as well as create scrapers. With this driver, you can load JavaScript on your website, which is not possible with modules like Scrapy, and Urllib. Conceptually you can imagine the Selenium driver to be the controller of your browser. In my experience, the most time-consuming part of Selenium coding is the setup of the web driver. Therefore, I have created an article about an easy way to setup the Selenium web driver. Any website that you visit will treat your driver as a browser and the driver will be able to load all dynamic elements that are dependent on JavaScript. I will describe two different kinds of “waits”:
- Explicit Wait
- Implicit Wait
Implicit Wait
An implicit wait in Selenium tells your driver to wait for a certain amount of time. You can specify the exact amount of time that your driver needs to wait. This is the most simple way of waiting in Selenium. However, it is not the recommended approach as it is not efficient and is error prone. For whatever reason, a certain element might take longer to load and your explicit wait might then nog be long enough. If you decide to play safe and increase the explicit wait duration, you risk writing a program that takes longer to execute than necessary. The syntax is as follows:
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
chromeDriverPath = '/driver/chromedriver'
chromeOptions = webdriver.ChromeOptions()
driver = webdriver.Chrome(chromeDriverPath, options=chromeOptions)
driver.get(url)
driver.implicitly_wait(20)
In this piece of code you are loading the driver using Chromium. Then you load a specific URL and after the load has started, you implicitly wait for 20 seconds. As mentioned above, this is not the recommended approach. Please read the explicit waits below for a more efficient approach.
Explicit wait
An explicit wait in Selenium tells your driver to wait for a specific expected condition. You can specify the exact amount of time that your driver needs to wait before an expected condition takes place. This expected condition could include amongst others, the visibility of an element, element clickable, text presence in an element. For more expected conditions you can check the Selenium Documentation.
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
chromeDriverPath = '/driver/chromedriver'
chromeOptions = webdriver.ChromeOptions()
driver = webdriver.Chrome(chromeDriverPath, options=chromeOptions) # Optional argument, if not specified will search path.
driver.get(url)
wait = WebDriverWait(driver, 20)
wait.until(EC.element_to_be_clickable((By.CSS_SELECTOR, {css_selector})))
As you can see we have specified a 20-second wait before a certain element is clickable. WebDriverWait() takes to arguments, the driver object, and the maximum number of seconds you want to wait. When we use wait.until we can specify the expected condition. The expected condition function then takes two arguments, one specifying how you want to search for that element, and then the value for the search. In our example, we are searching by CSS selectors and the second argument will take the actual CSS selector value, e.g. “body .title”. For more different types of search you can use with the By module you can check the following documentation.
I hope you have a better understanding of the different kinds of waits in Selenium. If you have any questions, do not hesitate to contact me.
Pingback: Easy Setup Selenium Webdriver - Automation Help
Pingback: Create a simple web scraper using Selenium - Automation Help