Are you trying to understand how to send HTTP requests using Google App Script? In this article I will dive deeper into how you can use one of the most powerful features of Google Sheet, the Google App Script editor. Whether you are a complete newcomer to the world of coding, or even if you have written many lines of codes, I will try to make this article understandable for anyone.
Google App Script
If you already know a little bit of JavaScript, writing Google App Script code should be really easy. Google has made it really easy for you to write your own JavaScript code and deploy it on the Google Servers. You have access to many different kinds of Google builtin libraries, which allow you to easily connect your code to services like Gmail, Google Drive, and Google Calendar. It will even allow you to publish web apps, create add-ons, and build chatbots.
The most relevant features for this article are the UrlFetchApp class that you are able to use, and the connection to Google Sheet to output your data. You can use Google App Script to send HTTP requests to an API, process the collected data, and export it in your Google Sheet.
Many different kind of HTTP exists, but we will focus on the two most important ones:
- GET requests
- POST requests
Get Requests
The GET request is the most simple HTTP request you can execute. This request only requires a URL and optionally a header that adds more information about your request.
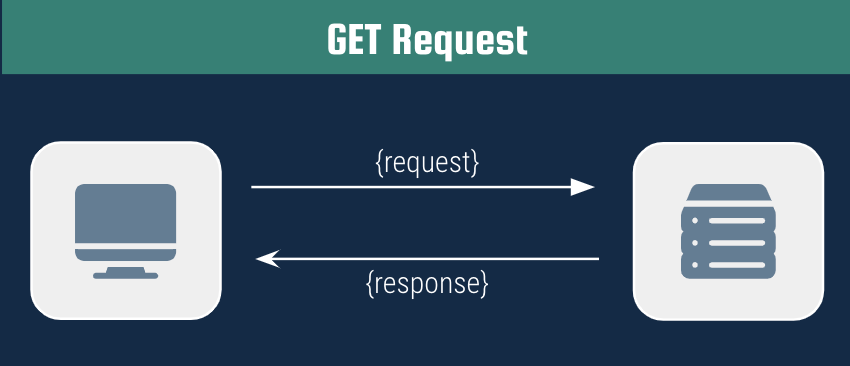
Within Google App Script you can send a GET request with the following lines of code. This assumes the response is a JSON object, and will append the response in a Sheet called “Data”.
function getRequest() {
// Make a Get request
var header = {
"user-agent": "Mozilla/5.0 (Linux; Android 6.0; Nexus 5 Build/MRA58N) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/81.0.4044.138 Mobile Safari/537.36"
};
var options = {
'method' : 'get',
'headers' : header
};
response = UrlFetchApp.fetch('URL', options);
console.log(response)
json_response = JSON.parse(response)
var ss = SpreadsheetApp.getActiveSpreadsheet(); //Get reference to active spreadsheet for when setting values in certain sheets;
var sheet = ss.getSheetByName('Data');
sheet.appendRow([json_response]);
POST Requests
The POST request is a bit more complicated HTTP request. This request requires a URL and a payload that contains data that you want to “post”. In this case you can also optionally add a header for more information about your request.
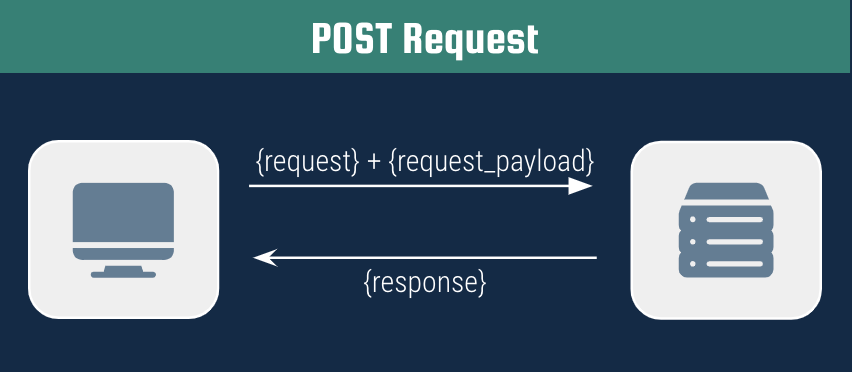
Within Google App Script you can send a POST request with the following lines of code. This assumes the response is a JSON object, and will append the response in a Sheet called “Data”.
function postRequest() {
// Make a POST request
var header = {
"user-agent": "Mozilla/5.0 (Linux; Android 6.0; Nexus 5 Build/MRA58N) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/81.0.4044.138 Mobile Safari/537.36"
};
var payload = {key: value}
var options = {
'method' : 'POST',
'headers' : header,
‘payload’: payload
};
response = UrlFetchApp.fetch('URL', options);
console.log(response)
json_response = JSON.parse(response)
var ss = SpreadsheetApp.getActiveSpreadsheet(); //Get reference to active spreadsheet for when setting values in certain sheets;
var sheet = ss.getSheetByName('Data');
sheet.appendRow([json_response]);
In both the GET as the POST request, we are using the UrlFetchApp class. For more information, you can check out Google’s Documentation. I hope this article has answered your question. If you need any help, do not hesitate to contact me.
Pingback: How to find email addresses - Automation Help
Thanks for this post.